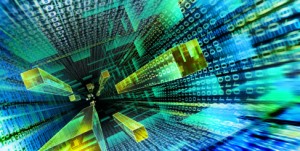
More fun with comments
I don’t know about you, but I find it a bit irritating clicking on a comment author’s link and being taken off site. So here’s a way to get the link to open in a new window.
If you read my previous post about customising the wp_list_comments() function, you will know that it isn’t so simple to access the elements that make up each comment output by this function.
Luckily, the WordPress developers have given a nice filter we can hook into, called get_comment_author_link. This means we can simply add a filter using this filter hook, rather than having to play aroung with wp_list_comments().
Writing a WordPress filter
Actually, the WordPress Plugin API gives you all the information you need but, in brief, here’s an overview. Filters are added to your theme’s functions.php in this format:
// Let's hook my function to a filter add_filter('filter_hook_name', 'function');
As you can see, the above code requires two things:
- filter_hook_name These are pre-defined in WordPress. In this example, we will use the get_comment_author_link filter hook.
- function This is the name of your custom function which will be used to “filter” the output of the filter_hook_name. Don’t worry too much about what this means, though you can read more in the API links posted above. In essence, it means that our function will hook into WordPress and enable us to modify the output of the chosen filter hook.
Writing the custom function
Here’s our function which will open the link in a new window:
// Make comment author link URL open in new window function comment_author_link_window() { global $comment; $url = get_comment_author_url(); $author = get_comment_author(); if ( empty( $url ) || 'http://' == $url ) $return = $author; else $return = "<a href='$url' rel='external nofollow' target='_blank'>$author</a>"; return $return; }
I’m not going to go into too much detail, other than to mention that our “custom” code can be found in line 10:
$return = "<a href='$url' rel='external nofollow' target='_blank'>$author</a>";
As you can see, it would be quite possible to remove the “nofollow” attribute too, should you wish. Did I mention how useful and poweful WordPress filters are?
Putting it all together
All we need do now is edit our add_filter code, mentioned above, to reference the correct filter hook name and the name of our new function. We can then add both snippets of code to the theme’s functions.php file, like so:
// Make comment author link URL open in new window function comment_author_link_window() { global $comment; $url = get_comment_author_url(); $author = get_comment_author(); if ( empty( $url ) || 'http://' == $url ) $return = $author; else $return = "<a href='$url' rel='external nofollow' target='_blank'>$author</a>"; return $return; } add_filter('get_comment_author_link', 'comment_author_link_window');
Enjoy!
Thanks for the info, you’ve enlightened my wordpress code knowledge a little bit. My wordpress templates aren’t exactly the same as yours, but I think I get the main point. I will go ahead and give it a try.
Great! 🙂
Gotta tell you, SO glad I found this. Works like a CHARM. More people need to read this page – I’ve been all over the net dude, and a LOT of people have this problem. It seems the most popular method is editing comment-template.php – granted, it works, but it gets overwritten every time you update WP (of course). I actually had 4 or 5 WP includes I’d edited to do specific things, but had to constantly scramble to fix the overrides on every WP update. I finally whittled it all down to comment-template.php with THIS problem, and I’ve been looking for methods to fix it without digging through the source anymore.
Anyway, to cut short my rambling – you just removed the final problem, and I can finally update WP freely and no longer worry. Thanks a million! You also opened up my eyes to functions.php capabilities! Very cool!
Damn, deleting this “WORDPRESS_EDITS” folder is gonna be crazy satisfying. Peace!
This is a great solution, I too have seen many template edit solutions, which is not optimal.
Since I use Hybrid template system, I modified the code code a bit, and added the ‘cite’ tag:
...
if ( empty( $url ) || 'http://' == $url )
$return = "$author";
else
$return = "$author";
return $return;
...
Thanks!
* look like the parser did not interpret the {code} tag correctly. Going to try again…
...
if ( empty( $url ) || 'http://' == $url )
$return = "{cite class='fn'}$author{/cite}";
else
$return = "{cite class='fn' title='$url'}{a href='$url' title='$author' class='url' rel='external nofollow' target='_blank'}$author{/a}{/cite}";
return $return;
Thanks for posting this, Henry. 🙂
Thank, dude! Appreciate your work, this works like a charm 🙂
Thank you alot for that Information.
In German here:
http://www.azella.de/blog/2011/08/wordpress-kommentarlinks-als-_blank/
Thanks for this. I will try this code on my blog sites.
Thanks for the code, it works like a charm!
What do you think about this way?
function drivingralle_edit_comment_author_link( $input ) {
// Add target=”_blank” to the link
$input = preg_replace(‘/(<a href[^]+)>/is’, ‘\\1 target=”_blank”>’, $input);
//return the modified string
return $input;
}
add_filter( ‘get_comment_author_link’, ‘drivingralle_edit_comment_author_link’ );
Yes, that should work, though there may be a slight performance hit thanks to preg_replace(). We’re talking about a very, very small hit though.